While analyzing the PerfView source code, I stumbled upon an interesting README file in the src/OSExtensions folder:
// The OSExtensions.DLL is a DLL that contains a small number of extensions
// to the operating system that allow it to do certain ETW operations.
//
// However this DLL is implemented using private OS APIs, and as such should
// really be considered part of the operating system (until such time as
// the OS provide the functionality in public APIs).
//
// To discourage taking dependencies on these internal details we do not
// provide the source code for this DLL in the open source repo.
//
// IF YOU SIMPLY WANT TO BUILD PERFIVEW YOU DO NOT NEED TO BUILD OSExtensions!
// A binary copy of this DLL is included in the TraceEvent\OSExtensions.
//***************************************************************************
// However we don't want this source code to be lost. So we check it in
// with the rest of the code but in an encrypted form for only those few
// OS developers who may need to update this interface. These people
// should have access to the password needed to unencrpt the file.
//
// As part of the build process for OSExtension.dll, we run the command 'syncEncrypted.exe'.
// This command keeps a encrypted and unencrypted version of a a file in sync.
// Currently it is run on this pair
//
// OSExtensions.cs <--> OSExtesions.cs.crypt
//
// Using a password file 'password.txt'
//
// Thus if the password.txt exists and OSExtesions.cs.crypt exist, it will
// unencrypt it to OSExtesions.cs. If OSExtesions.cs is newer, it will
// be reencrypted to OSExtesions.cs.crypt.
Hmm, private OS APIs seem pretty exciting, right? A simple way to check these APIs would be to disassemble the OSExtensions.dll (for example, with dnSpy). But this method would not show us comments. And for internal APIs, they might contain valuable information. So let’s see if we can do better.
How OSExtensions.cs.crypt is encrypted
As mentioned in the README, internal PerfView developers should use the provided password.txt file and the SyncEncrypted.exe application. The SyncEncrypted.exe binaries are in the same folder as OSExtensions.cs.crypt, and they are not obfuscated. So we could see what’s the encryption method in use. The Decrypt
method disassembled by dnSpy looks as follows:
// Token: 0x06000003 RID: 3 RVA: 0x000022A8 File Offset: 0x000004A8
private static void Decrypt(string inFileName, string outFileName, string password)
{
Console.WriteLine("Decrypting {0} -> {1}", inFileName, outFileName);
using (FileStream fileStream = new FileStream(inFileName, FileMode.Open, FileAccess.Read))
{
using (FileStream fileStream2 = new FileStream(outFileName, FileMode.Create, FileAccess.Write))
{
using (CryptoStream cryptoStream = new CryptoStream(fileStream, new DESCryptoServiceProvider
{
Key = Program.GetKey(password),
IV = Program.GetKey(password)
}.CreateDecryptor(), CryptoStreamMode.Read))
{
cryptoStream.CopyTo(fileStream2);
}
}
}
}
You don’t see DES being used nowadays as its key length (56-bit) is too short for secure communication. However, 56-bit key space contains around 7,2 x 1016 keys, which may be nothing for NSA but, on my desktop, I wouldn’t finish the decryption in my lifetime. As you’re reading this post, you may assume I found another way :). The key to the shortcut is the Program.GetKey
method:
// Token: 0x06000004 RID: 4 RVA: 0x0000235C File Offset: 0x0000055C
private static byte[] GetKey(string password)
{
return Encoding.ASCII.GetBytes(password.GetHashCode().ToString("x").PadLeft(8, '0'));
}
The code above produces up to to 232 (4 294 967 296) unique keys, which is also the number of possible hash codes. And attacking such a key space is possible even on my desktop.
Preparing the decryption process
Now, it’s time to decide what we should call successful decryption. Firstly, it must not throw an exception, so the padding must be valid. As the padding is not explicitly set, DES will use PKCS7. Also, the mode for operation will be CBC:
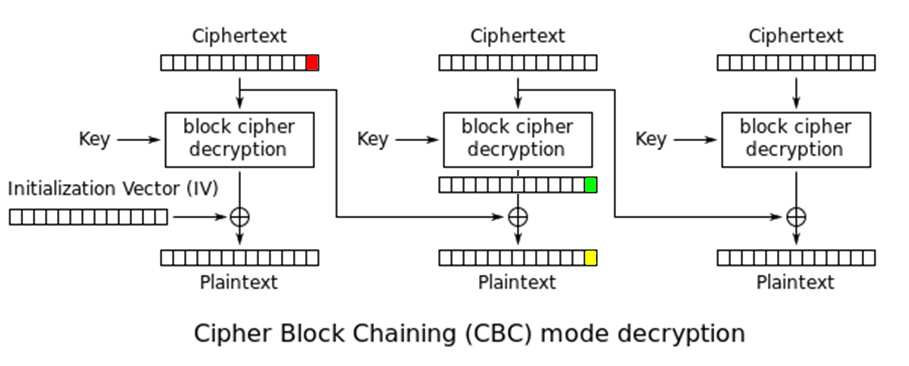
We also know that the resulting C# file should contain mostly readable text, so it should have a high percentage of bytes in the ASCII range (assuming the data is UTF-8 encoded). Checking all these conditions would work best if we were trying to decrypt the whole file in each try. However, doing so would consume much time as the file is about 40 KB in size. An improvement would be to use the first few blocks of the encrypted file for counting the ASCII statistics, and two last blocks for the padding validation. Fortunately, we can do even better. I noticed that all the source code files in the repository are UTF-8 encoded with BOM. That means we could try decrypting only the first 64 bits of the ciphertext and check if the resulting plaintext starts with 0xEF
, 0xBB
, 0xBF
. If it does, it may be the plaintext we are looking for. By appending the last two blocks of the ciphertext, we could also validate the padding. I haven’t done that, and I just disabled padding in DES. My decryptor code in F# (I’m still learning it) looks as follows:
open System
open System.IO
open System.Reflection
open System.Security.Cryptography
open System.Text
open System.Threading
let chars = "0123456789abcdef"B
[<Literal>]
let PassLen = 8
let homePath = Path.GetDirectoryName(Assembly.GetExecutingAssembly().Location)
let decrypt (des : DESCryptoServiceProvider) (cipher : byte array) (plain : byte array) (key : byte array) =
use instream = new MemoryStream(cipher)
use outstream = new MemoryStream(plain)
use decryptor = des.CreateDecryptor(key, key)
use cryptostream = new CryptoStream(instream, decryptor, CryptoStreamMode.Read)
cryptostream.CopyTo(outstream)
let rec gen n (s : byte array) = seq {
for i = 0 to chars.Length - 1 do
if n = s.Length - 1 then
s.[n] <- chars.[i]
yield s
else
s.[n] <- chars.[i]
yield! gen (n + 1) s
s.[n + 1] <- chars.[0]
}
let cipher = File.ReadAllBytes(Path.Combine(homePath, @"OSExtensions.cs.crypt"))
let tryDecrypt (state : byte array) =
let checkIfValid (plain : byte array) =
plain.[0] = byte(0xef) && plain.[1] = byte(0xbb) && plain.[2] = byte(0xbf)
// no padding so we won't throw unnecessary exceptions
use des = new DESCryptoServiceProvider(Padding = PaddingMode.None)
let plain = Array.create PassLen 0uy
// we will generate all random strings starting from the second place in the string
for pass in (gen 1 state) do
decrypt des cipher plain pass
if checkIfValid plain then
let fileName = sprintf "pass_%d_%d.txt" Thread.CurrentThread.ManagedThreadId DateTime.UtcNow.Ticks
File.WriteAllLines(Path.Combine(homePath, fileName), [|
sprintf "Found pass: %s" (Encoding.ASCII.GetString(pass));
sprintf "Decrypted: %s" (Encoding.ASCII.GetString(plain))
|])
[<EntryPoint>]
let main argv =
let zeroArray = Array.create (PassLen - 1) '0'B
chars |> Array.map (fun ch -> async { zeroArray |> Array.append [| ch |] |> tryDecrypt })
|> Async.Parallel
|> Async.RunSynchronously
|> ignore
0 // return an integer exit code
It’s also published in my blog samples repository.
And the content of OSExtensions.cs.crypt is:
0 1 2 3 4 5 6 7 8 9 A B C D E F 0000: 7b 37 a0 49 50 0b b2 49 {7.IP..I
Decrypting the file
After about 40 minutes, the application generated 108 pass_ files already and one of them had the following content:
Found pass: 436886a4 Decrypted: ???using
The using
statement is a very probable beginning of the C# file :). And indeed, the hash code above decrypts the whole OSExtensions.cs.crypt file. We won’t know the original PerfView password, but, as an exercise, you may try to look for strings that have the above hash code. If you find one, please leave a comment!
You might can use hashcat https://hashcat.net/hashcat/. It’s way fast, afaik has dictionaries and can use your GPU for faster brute force.
Thanks for the interesting idea! I have heard of hashcat but never really used it.